Page 2
Timing an Event in DataFlex
by Curtis Krauskopf
How Long
Did that Take?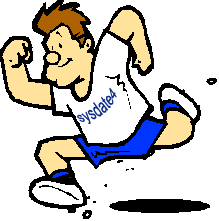
Both
SYSDATE and SYSDATE4 work well if you only want a rough
estimate of how long an event takes. The most common
technique for timing an event is:
- Use
SYSDATE4 to record the time from the system clock
- Let
the event happen
- Use
SYSDATE4 to record the system clock's time after the
event
This
example program shows how to use SYSDATE4 to find out
how long an event takes:
// howbored.src
// by Curtis Krauskopf at www.decompile.com
//
// This is an example of timing how long an event takes.
//
date before after
integer before_hour before_minute before_second
integer after_hour after_minute after_second
sysdate4 before before_hour before_minute before_second
showln "The clock has started ticking."
showln "When you get bored, press any key."
showln "..."
string akey 1
inkey akey
sysdate4 after after_hour after_minute after_second
number before_time // Accumulated number of seconds
number after_time
move (integer(before) * 3600 * 24) to before_time
move (before_time + (before_hour * 3600)) to before_time
move (before_time + (before_minute * 60)) to before_time
move (before_time + (before_second)) to before_time
move (integer(after) * 3600 * 24) to after_time
move (after_time + (after_hour * 3600)) to after_time
move (after_time + (after_minute * 60)) to after_time
move (after_time + (after_second)) to after_time
show "It took you " (after_time - before_time) showln " seconds to become bored."
showln
showln "Press any key to continue."
inkey akey
|
The
output of the program looks like this:
This
algorithm works even when the event starts on one day
and ends on the next day (across midnight).
A
DataFlex date is an integer that represents the number
of days since the hypothetical date: January 0, Year
0. It's almost like a Julian date, but not quite. A
Julian date is based on January 1, 4713 BC whereas a
DataFlex date is based on January 1, Year 0. The
United States Navy has an interesting site that
discusses Julian dates and its history in much greater
detail.
The
line
move (integer(before) * 3600 * 24) to before_time
|
converts
the date called before into seconds. The rest
of that block of code:
move (before_time + (before_hour * 3600)) to before_time
move (before_time + (before_minute * 60)) to before_time
move (before_time + (before_second)) to before_time
|
adds
the number of seconds in the hours, minutes and seconds
to the before_time accumulator.
Likewise,
the next block of code calculates the number of seconds
for all of the after_* variables.
Next,
the (after_time -
before_time) expression calculates
the difference in seconds.
When
playing with this program, notice a couple things:
When
the time delay is very short, you might see:
or
The
first result is wrong because it takes at least some
time to 'become bored', even if it's only a fraction
of a second.
The
second result is wrong if you became bored in less than
one second but the computer reports that it took you
an entire second to become bored.
A
similar problem exists for longer delays too. Using
a stopwatch, compare how long the program reports that
you took versus how long you actually take. It seems
like the time reported by the computer is off by as
much as one second. And this really is the case.
Copyright
2003 The Database Managers, Inc. |